The Dark Side Of Software: Anti-Patterns (and How To Fix Them)
Behind every buggy app lies an Anti-Pattern waiting to be uncovered.
Introduction
An Anti-Pattern is a proven way to "shoot yourself in the foot." The term Anti-Pattern was coined by Andrew Koenig and it's pretty entertaining to read about it in the "Design Patterns: Elements of Reusable Object-Oriented Software", published in 1994. The author defines Anti-Patterns as a "commonly-used process, structure or pattern of action that, despite initially appearing to be an appropriate and effective "Response to a problem, has more bad consequences than good ones."
In 1998 the term "Anti-Pattern" became popular thanks to the book "AntiPatterns: Refactoring Software, Architectures, and Projects in Crisis". It defined Anti-Patterns as "specific repeated practices in software architecture, software design, and software project management that initially appear to be beneficial, but ultimately result in bad consequences that outweigh hopes-for advantages."
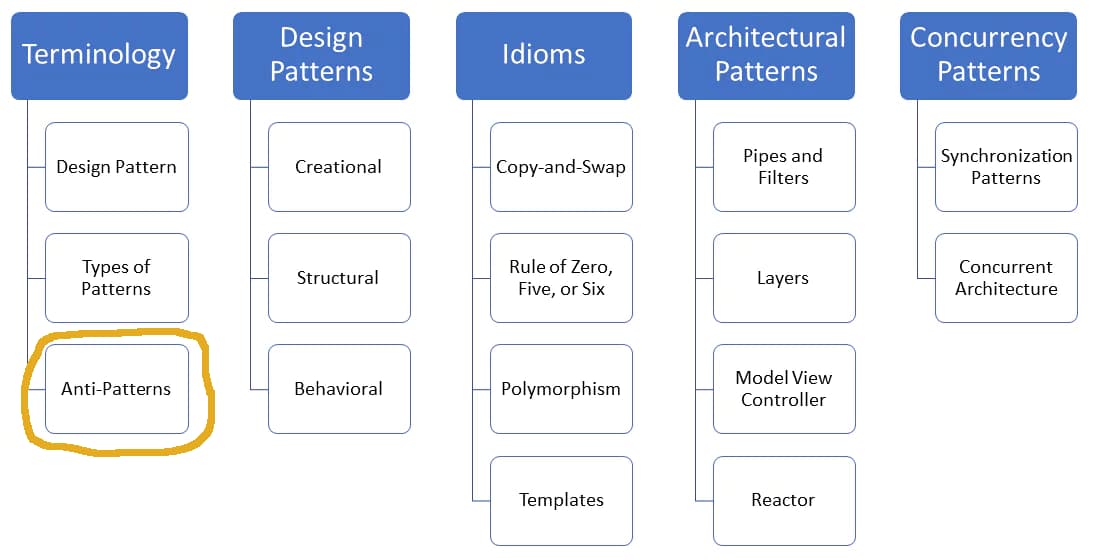
In the following article, we will learn about the reason why Anti-Patterns are used, the most common Anti-Patterns, and possible solutions that can be used to avoid these Anti-Patterns.
Reasons Anti-Patterns are used
An Anti-Pattern, similar to a design pattern, is a literary form and simplifies the communication and description of common problems. Often Anti-Patterns are a design pattern applied in the wrong context.
In Software Development, the following factors are often the main causes of Anti-Patterns.
- Pressure of time
- Disinterest
- Closed-Mindedness
- Laziness
- Stinginess
- Ignorance
- Pride
Countermeasures To Avoid Anti-Patterns
To counter these factors, software design and development must take the following fundamental forces into account when making decisions about the project:
- Functionality Management
- Ensure that the product delivers the features while balancing between scope and feasibility.
- Complexity Management
- Simplify design and solutions to reduce unnecessary complexity of the software. Also, improve maintainability.
- Performance Management
- Address non-functional requirements like development speed, reliability, and scalability to improve quality.
- Change Management
- Adopt a flexible strategy to handle new requirements and unexpected changes without restarting the project.
- IT Resource Management
- Allocate and utilize all technical resources efficiently.
- Technology Transfer Management
- Plan the adoption of new technologies and ensure smooth integration with existing systems.
Domains of Anti-Patterns
In the previously mentioned book "AntiPatterns: Refactoring Software, Architectures, and Projects in Crisis" the Anti-Patterns are categorized into three different domains which cover most of the common areas where Anti-Pattern can be seen. However, nowadays, additional perspectives and domains were identified or created which also can have different Anti-Patterns.
Software Development: This domain focuses on coding and implementation practices and its Anti-Patterns lead to poor software structure which makes it harder to maintain and extend.
Software Architecture: This domain is about system- and enterprise-level design which often leads to faulty or rigid architectures in the system. This avoids scalability and adaptability for further development.
Software Project Management: This is all about team coordination, resource allocation, and timelines. Often brought about by poor communication and bad leadership resulting in chaos and inefficiency.
Software Operations and Deployment: Anti-Patterns in this domain involve the deployment, monitoring, and scaling of software in production environments.
User Experience (UX) and Design: This domain describes the usability and accessibility of software in which ignoring UX often leads to not meeting user expectations.
Team Dynamics and Culture: This domain focuses on the human side of software development, including collaboration and morale. If not maintained it creates a toxic culture and poor team dynamics which can fail even well-structured projects.
Security and Compliance: In this domain security vulnerabilities and adherence to regulatory requirements are addressed because ignoring best practices or compliance requirements can result in security breaches or fines for the company.
Anti-Patterns In Software Development
The God Object
🙌 Support this content
If you like this content, please consider supporting me. You can share it on social media, buy me a coffee, or become a paid member. Any support helps.
See the contribute page for all (free or paid) ways to say thank you!
Thanks! 🥰